· 4 min read
React Custom Hook: usePrevious
The usePrevious hook is a custom React hook that allows you to access the previous value of a state or prop.
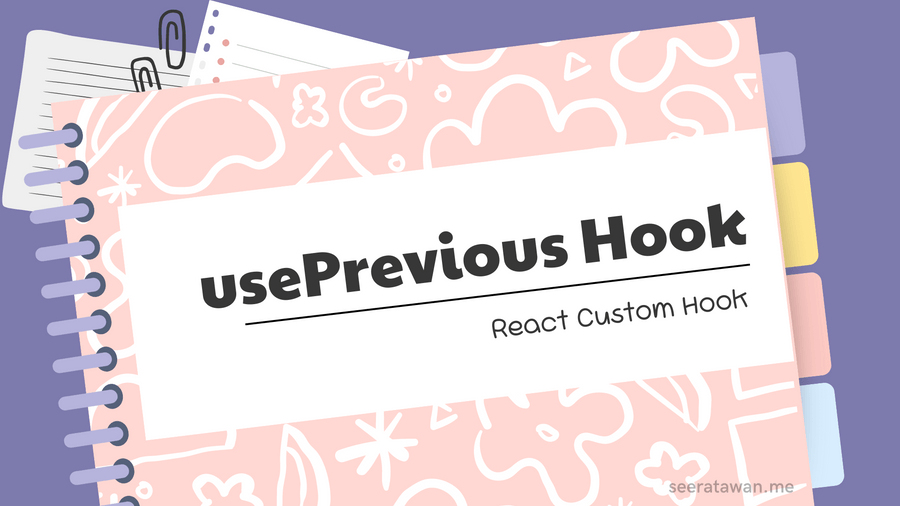
React hooks have revolutionized the way we develop components and manage state in React applications. While React provides several built-in hooks, such as useState
and useEffect
, sometimes you may need more specialized functionality tailored to your application’s needs.
This is where custom hooks come into play, and one particularly useful hook is the
usePrevious
hook.
What is the usePrevious
Hook?
The usePrevious
hook is a custom React hook that allows you to access the previous value of a state or prop. It can be incredibly useful in scenarios where you need to compare the current value with the previous value or perform side effects based on value changes.
For example, you might want to trigger an animation when a value changes, log the previous value for debugging purposes, or optimize performance by skipping expensive computations if the value hasn’t changed.
Implementation
Here’s the implementation of the usePrevious
hook:
import { useEffect, useRef } from 'react';
export function usePrevious<T>(value: T): T | undefined {
const ref = useRef<T>();
useEffect(() => {
ref.current = value;
}, [value]);
return ref.current;
}
Let’s break down the code:
- Importing Dependencies: First, we import the
useEffect
anduseRef
hooks from the React library. - Hook Definition: The
usePrevious
function is defined as a generic function, allowing it to work with any data type (T
). It takes avalue
parameter of typeT
and returns eitherT
orundefined
. - Creating a Mutable Reference: The
useRef
hook is used to create a mutable reference (ref
) that persists across component re-renders. The initial value ofref.current
isundefined
. - Updating the Reference: An
useEffect
hook is used to update theref.current
value whenever thevalue
changes. The empty dependency array[value]
ensures that the effect is re-executed whenevervalue
changes. - Returning the Previous Value: The hook returns the current value of
ref.current
, which will be the previous value ofvalue
after the component re-renders.
Usage
Using the usePrevious
hook is straightforward. Here’s an example:
import { useState } from 'react';
import { usePrevious } from './usePrevious';
function Counter() {
const [count, setCount] = useState(0);
const previousCount = usePrevious(count);
return (
<div>
<h1>Current Count: {count}</h1>
{previousCount !== undefined && (
<p>Previous Count: {previousCount}</p>
)}
<button onClick={() => setCount(count + 1)}>Increment</button>
</div>
);
}
In this example, we import the usePrevious
hook and use it to store the previous value of the count
state. When the “Increment” button is clicked, the count
state is updated, and the previous value of count
is displayed below the current count.
Benefits
Using the usePrevious
hook can provide several benefits in your React applications:
- State Comparison: By having access to the previous value of a state or prop, you can easily compare it with the current value and perform conditional logic or side effects based on the changes.
- Side Effect Handling: The
usePrevious
hook can be particularly useful when you need to perform side effects based on value changes, such as triggering animations, logging changes, or making API calls. - Performance Optimization: In certain cases, you can use the
usePrevious
hook to skip unnecessary computations or re-renders if the value hasn’t changed, improving the overall performance of your application. - Debugging: Having access to the previous value can be helpful for debugging purposes, especially when tracking down issues related to state changes or side effects.
Conclusion
The usePrevious
custom hook is a powerful tool in the React developer’s toolkit. By allowing you to access the previous value of a state or prop, it enables efficient state management, side effect handling, and performance optimizations. Whether you’re building complex user interfaces, implementing animations, or optimizing performance, the usePrevious
hook can prove invaluable in streamlining your React development process.
Embrace the power of custom hooks and take your React development skills to the next level with the usePrevious
hook. Unlock new possibilities and create more efficient and responsive applications today!
Need a React Developer? Let’s Work Together!
Are you impressed by the usePrevious hook and looking for an experienced React developer to bring your project to life?
Feel free to reach out to me here - Contact Seerat Awan to discuss your project and get a quote.
With a deep understanding of React and other javascript based frameworks, I can help you build high-performance, efficient, and user-friendly applications tailored to your specific needs.
Don’t hesitate to get in touch – I’m excited to hear about your project and explore how we can collaborate to create exceptional React applications together!
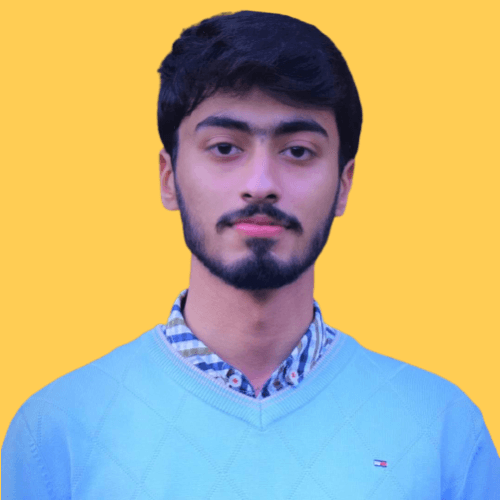
Subscribe to my newsletter to get the latest updates on my blog.