· 4 min read
React Custom Hook: useDidMountEffect
The useDidMountEffect hook in React allows handling side effects after the initial render conveniently.
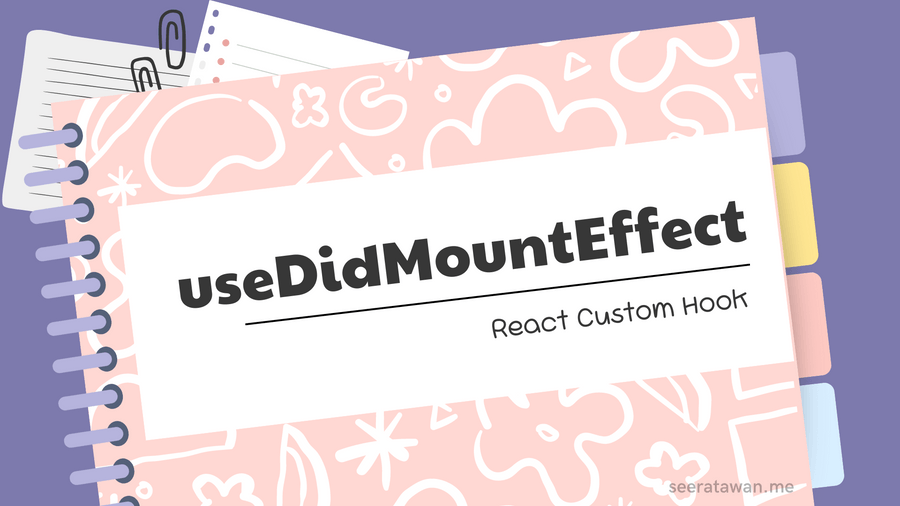
In the world of React development, managing side effects is a crucial aspect of building efficient and responsive applications. While the built-in useEffect
hook is a powerful tool for handling side effects, there are cases where you may want to skip the effect during the initial render and only execute it on subsequent updates based on specific dependencies.
This is where the
useDidMountEffect
hook comes into play. This custom hook is designed to provide a more precise control over when side effects are executed, helping you optimize your React components and improve their performance.
Implementation
Here’s the implementation of the useDidMountEffect
hook:
import { useEffect, useRef } from 'react';
export function useDidMountEffect(effect: () => any, deps : Array<any>) {
const didMount = useRef(false);
useEffect(() => {
if (didMount.current) {
return effect();
} else {
didMount.current = true;
}
}, deps);
}
Let’s break down the code:
- Importing Dependencies: The hook imports the
useEffect
anduseRef
hooks from the React library. - Hook Definition: The
useDidMountEffect
function is defined, taking two parameters:effect
(the side effect function to be executed) anddeps
(an optional array of dependencies, similar touseEffect
). - Mutable Reference: The
useRef
hook is used to create a mutable referencedidMount
, which is initially set tofalse
. - Effect Implementation: An
useEffect
hook is used to handle the side effect. Inside the effect callback, a check is performed ondidMount.current
. If it’strue
, theeffect
function is executed, and its cleanup function (if any) is returned. IfdidMount.current
isfalse
, it’s set totrue
, effectively skipping the side effect on the initial render. - Dependency Array: The
deps
array is passed as the second argument to theuseEffect
hook, ensuring that the effect is re-executed whenever any of the dependencies change.
Usage
Using the useDidMountEffect
hook is similar to using the standard useEffect
hook. Here’s an example:
import { useState } from 'react';
import { useDidMountEffect } from './useDidMountEffect';
function Counter() {
const [count, setCount] = useState(0);
useDidMountEffect(() => {
console.log('Count changed:', count);
}, [count]);
return (
<div>
<h1>Count: {count}</h1>
<button onClick={() => setCount(count + 1)}>Increment</button>
</div>
);
}
In this example, we import the useDidMountEffect
hook and use it to log the current value of the count
state whenever it changes. However, unlike the standard useEffect
hook, the logging effect will not be executed during the initial render of the component. It will only be triggered on subsequent updates when the count
state changes.
Benefits
Using the useDidMountEffect
hook can provide several benefits in your React applications:
- Precise Effect Handling: The hook allows you to skip side effects during the initial render and only execute them on subsequent updates, providing more precise control over effect handling.
- Performance Optimization: By avoiding unnecessary side effects during the initial render, you can potentially improve the performance of your components, especially in cases where the side effect involves expensive computations or API calls.
- Improved Code Readability: By separating the initial render logic from the update logic, your code can become more readable and maintainable, making it easier to understand and reason about the component’s behavior.
- Flexibility: The
useDidMountEffect
hook maintains the same API as the standarduseEffect
hook, allowing you to leverage the familiar dependency array for controlling when the effect should be re-executed.
Conclusion
The useDidMountEffect
hook is a valuable addition to your React development toolkit, providing a specialized solution for precise side effect handling in your components. By allowing you to skip effects during the initial render and execute them only on subsequent updates based on specific dependencies, this hook can help you optimize performance and improve code readability.
Whether you’re building complex user interfaces, implementing performance-critical features, or simply seeking to enhance the maintainability of your React codebase, the useDidMountEffect
hook can be a powerful ally. Embrace the power of custom hooks and take your React development skills to new heights!
Need a React Developer? Let’s Work Together!
Are you impressed by the usePrevious hook and looking for an experienced React developer to bring your project to life?
Feel free to reach out to me here - Contact Seerat Awan to discuss your project and get a quote.
With a deep understanding of React and other javascript based frameworks, I can help you build high-performance, efficient, and user-friendly applications tailored to your specific needs.
Don’t hesitate to get in touch – I’m excited to hear about your project and explore how we can collaborate to create exceptional React applications together!
- react
- custom-hooks
- usedidmounteffect
- side-effects
- performance-optimization
- react-hooks
- javascript
- webdevelopment
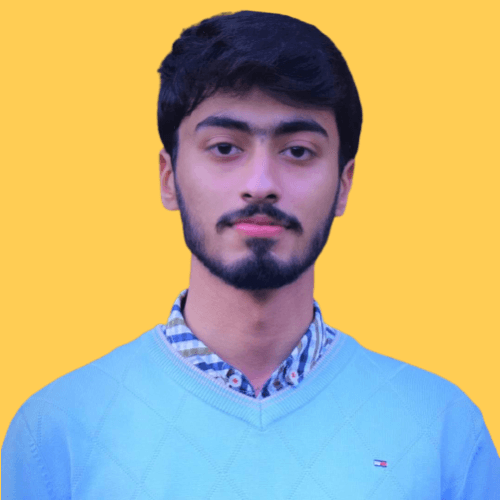
Subscribe to my newsletter to get the latest updates on my blog.